Overview
Morse in One is a npm package for morse code. It has many functionality like encoding sentences in morse code. The `mcConvert` function can convert sentences into morse code in linear time.
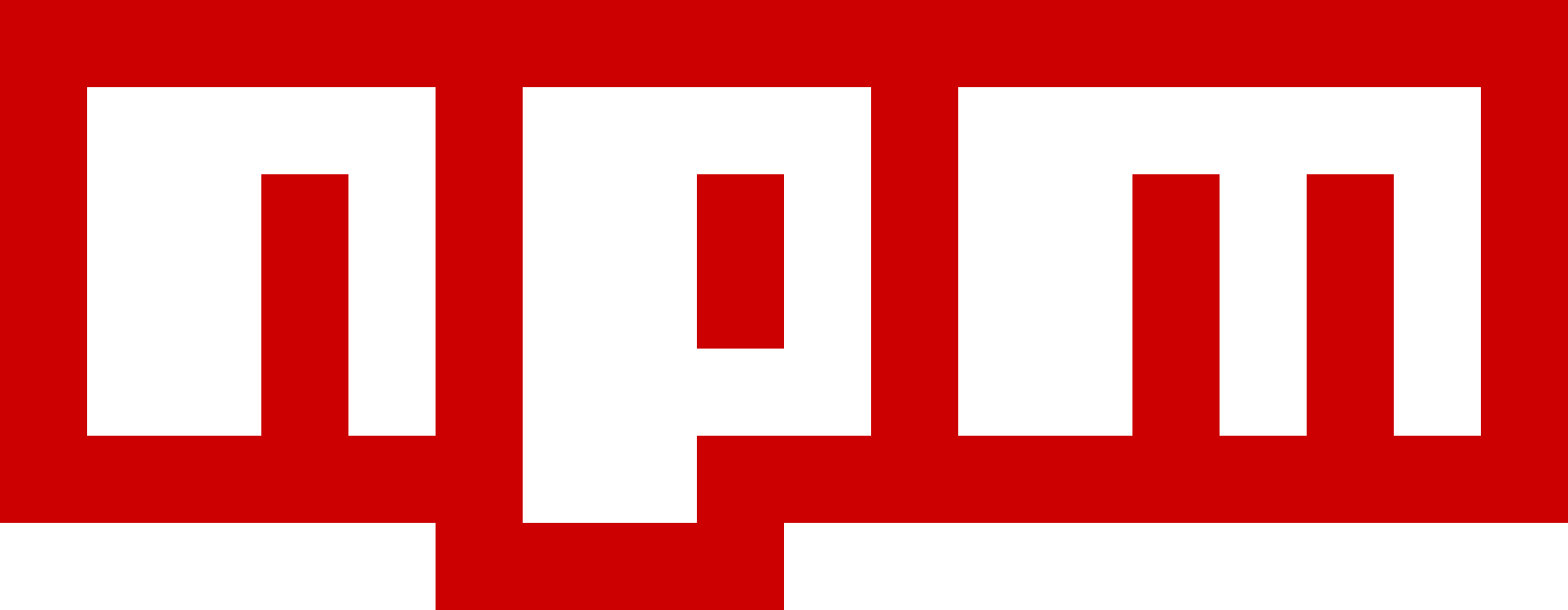
Installation
Assuming that NodeJs and npm or any package manager are installed in your system. Make a project directory in your system.
$ mkdir project
$ cd project
Then initialize your package
$ npm init
If you are using npm :
$ npm install morse-in-one
If you are using yarn :
$ yarn add morse-in-one
Encode Morse
It has many functionality like encoding sentences in morse code. Thee `mcConvert` function can convert sentences into morse code in linear time.
import { mcConvert } from 'morse-in-one';
console.log(mcConvert('hello world!'));
Output :
.... . .-.. .-.. --- / .-- --- .-. .-.. -.. -.-.--
Decode Morse
This package has a morse decoder function `mcDecode`, it decodes morse code into English.
import { mcDecode } from 'morse-in-one';
console.log(mcDecode('.... . .-.. .-.. ---'));
Output :
hello
Class Object
MorseCode class has more functionality with clean code.
import { MorseCode } from 'morse-in-one';
const obj = new MorseCode('Hi, I am a Developer')
console.log(obj.mcCode)
Output :
.... .. --..-- / .. / .- -- / .- / -.. . ...- . .-.. --- .--. . .-.
MorseCode Class Attributes :
Attribute Name | Description |
---|---|
realStr | Real input string |
mcCode | Morse code of input string |
strLen | Length of input string |
mcLen | Length of morse code of input string |
strLen
import { MorseCode } from 'morse-in-one';
const newObj = new MorseCode('hi');
console.log(newObj.strLen)
Output :
2
mcLen
console.log(newObj.mcLen)//.... ..
Output :
7
mcCode
console.log(newObj.mcCode)//.... ..
Output :
.... ..
realStr
console.log(newObj.realStr)//hi
Output :
hi
MorseCode Class Methods :
Methods Name | Description | Parameteres |
---|---|---|
mcConvert() | Encode into morse code | None |
mcDecode() | Decode morse code into English | None |
calcStrLen() | Return length of input string | None |
calcMcLen() | Return length of morse string | None |
toJson() | Create a JSON file with inputStr and outputCode keys. | Single parameter : file name |
toTxt() | Create a TXT file with morse code text. | Single parameter : file name |
To Json File Export
This function takes two parameter, first is input string and a file name string and create a JSON file with "inputStr" and "outputCode" keys.
import { toJson } from 'morse-in-one';
const str = hello
toJson(str,'<file-name>');
Output :
JSON file { "inputStr": "hello", "outputCode": ".... . .-.. .-.. --- / .-- --- .-. .-.. -.. -.-.--" }
To Txt File Export
This function takes two parameter, first is input string and a file name string and create a TXT file with morse code of input string.
import { toTxt } from 'morse-in-one';
const str = hello
toTxt(str,'<file-name>');
Output :
TXT file contains .... . .-.. .-.. --- / .-- --- .-. .-.. -.. -.-.--